Status codes, the status of a p[age being requested from a web server can be generated in a number of different ways. Here we show you how this is done in PHP code – a language that can be used to generate HTML web pages directly.
- Why use PHP to generate status codes?
- What are Status Codes?
- HTTP Status Code Types - Overview
- PHP - An Overview
- Returning A Status Code In PHP
- Example: Return a 400 bad request status code
- Example: Return a 404 not found status code
- Example: Return a 301 moved permanently status code
- Why Status Codes Matters For SEO
- Further Reading
Discover how SISTRIX can be used to improve your search marketing. 14 day free, no-commitment trial with all data and tools: Test SISTRIX for free
When browsing the internet as a user, you are probably unaware of the secret messaging that is being sent back and forth between where the website is being hosted and your browser.
For example, domain names are actually a series of numerical combinations. Status codes are similar in that they give information about if a page has loaded successfully or not, and the root cause of any errors. PHP is a scripting language that can generate status-code data.
While your content management system, possibly (WordPress) and your web server (possibly Apache) can generate these codes, the scripting language PHP, which is the basis of WordPress, can also generate these codes.
Why use PHP to generate status codes?
PHP is the language that WordPress is built on. If you are thinking of adapting your WordPress theme, or even writing additional pages using PHP, you might want to use status codes to return a positive status code, to redirect the request to another page or site, or to advise that a page is not available. For example, you have deleted a lot of content and you want to provide a special landing page to tell robots and users that this specific content has been removed, and why. Or, you may want to write a simple PHP script to tell users when the site is under maintenance.
What are Status Codes?
HTTP status codes are a way that servers communicate with clients (browsers). For the most part, the page loads successfully and so an ‘ok’ 2xx code will be generated. In such a case, status codes remain invisible to the user. But status codes are there to cover all eventualities, such as a 404 error or even a 502 bad gateway, which will be visually displayed on the page as an error message.
Understanding status codes will allow you to enhance your user experience, especially if your website visitors are receiving error codes that originate from your server as an example.
As the practice is quite technical, status codes are usually implemented manually by someone who understands coding such as a web developer. However, if your website is on WordPress, then plugins do exist to help you make sense and implement status codes.
Of course, as a website user, you may also come across status codes on other websites too. For example, 403 forbidden can be generated if you try to access a section of a website that you don’t have permission to view.
HTTP Status Code Types – Overview
- 1xx informational response
- 2xx success
- 3xx redirection
- 4xx client errors
- 5xx server errors
- Unofficial status codes
More detail is available in our http status code overview article.
PHP – An Overview
PHP stands for hypertext preprocessor. As you may have noticed, the acronym is a little confusing and that’s because PHP originally stood for personal home page. Remember, the way we develop websites has changed immensely in the short time the internet has existed, so sometimes terms need to be updated to keep up to modern standards.
Whenever you request a URL, a complex chain occurs between the server and the browser. PHP is usually involved in this process, as it’s responsible for interpreting the data. A common example of where you would see PHP in action is a login page. As you enter your credentials, a request to the server is made, and PHP will communicate with the database to log you in.
Essentially, PHP is a scripting language that is embedded into HTML. For a quick example, left click on any webpage and select ‘view page source’. Doing so will bring up the code that makes up that page. It is your browser that interprets the code into the functional version of the website.
With PHP, code can either be processed client side (HTML, Javascript and CSS) or server side (PHP). In essence, the server side of PHP is software that is installed on the server. This software can include Linux, Apache, MySQL and finally PHP. In that order, these 4 elements make up what’s known as a LAMP stack. The PHP is the scripting layer of this combination which websites and web applications run off.
Returning A Status Code In PHP
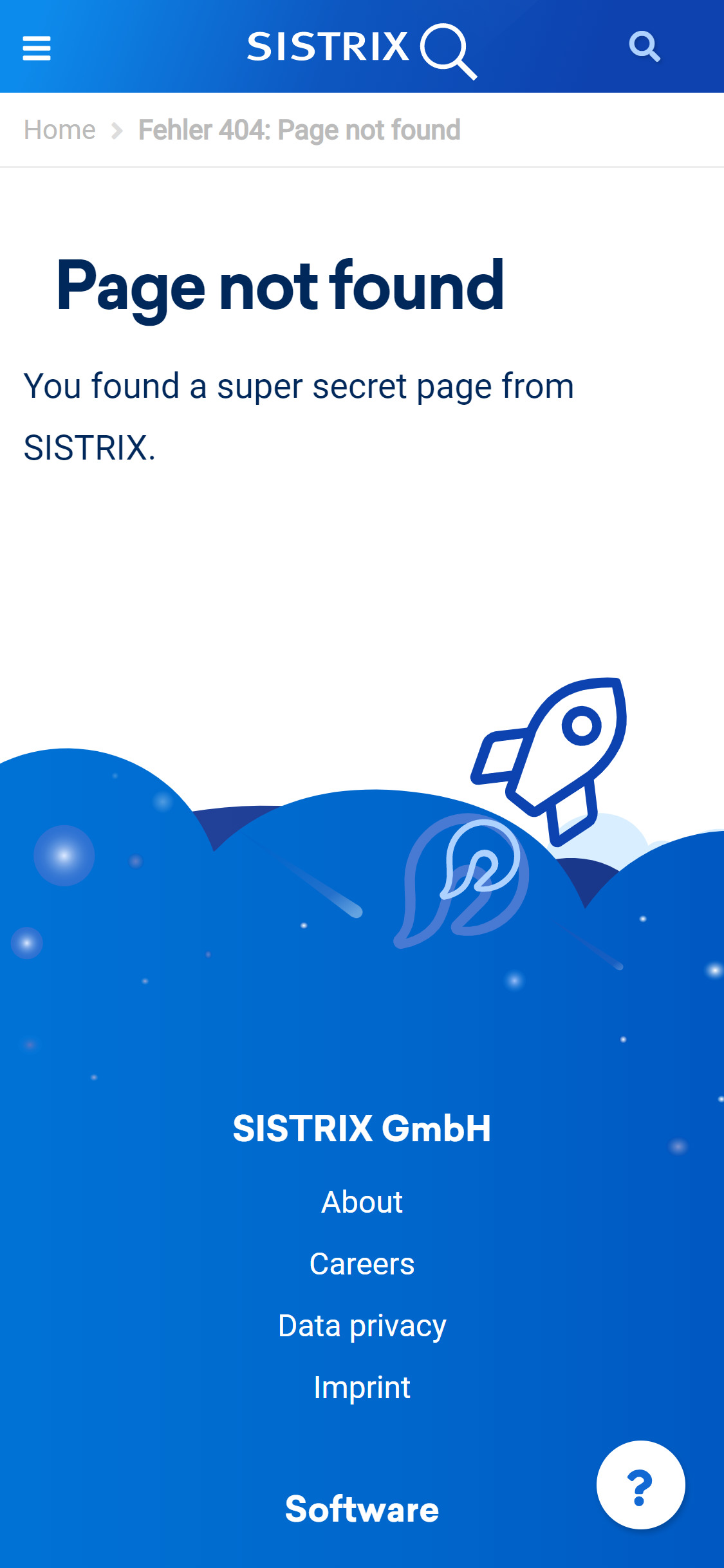
To return a status code in PHP, the simplest way is to use the http_response_code() function, along with the relevant HTTP status code parameter into your website, followed by the exit() command which stops any more output being set.
This means the likes of 400 bad requests and 404 not found can all be implemented with just one line of code.
Important: The http_response_code and header() commands must be used before any HTML is returned.
Example: Return a 400 bad request status code
http_response_code(400);
exit;
Example: Return a 404 not found status code
http_response_code(404);
exit;
Example: Return a 301 moved permanently status code
This example php code also requires that you provide the new URL, to which the users browser will automatically redirect. For this you need to use the more details header() function.
http_response_code(301);
header('Location: /newlocation.html');
exit;
The exit command means that no other php code or html code will be output from the page.
Here’s example code for a 503 temporary error page that also includes additional information that is shown in the browser.
<?php
http_response_code(503);
header( 'Retry-After: 600' );
?>
<!DOCTYPE html>
<html>
<head>
<title>php-generated 503</title>
</head>
<body>
This is a 503 error page.<br/> The error is returned in the HTTP header and this is just simple HTML that is displayed in the browser.
</body>
</html>
Why Status Codes Matters For SEO
As the name suggests, SEO is all about catering to search engines, so that users are more likely to come across your site. Search engines actively crawl status codes and will determine how your site is indexed as a result.
For example, if your site has plenty of 404 errors that exist from internal or external, links then this can harm your rankings because this will not generate a helpful experience for users. In a nutshell, search engines are looking out for healthy status codes, as this indicates everything is ticking over as it should be.
Further Reading
The above gives a brief overview of returning status codes in PHP. However, given the complex nature of coding it’s impossible to cover everything in just one article alone. So we definitely suggest doing some further reading to increase your understanding.
Resources you may find helpful include the official PHP website. In particular, their Using PHP section covers common errors you may encounter, especially as you get to grips with it.
Remember, when building any code it’s essential to test it. Even a small error or even a bug can disrupt the final result, so it’s good to remember that PHP isn’t just about the writing of the script, but seeing it through to a working page. Plus, looking out for any errors that may occur further down the line.
Test SISTRIX for Free
- Free 14-day test account
- Non-binding. No termination necessary
- Personalised on-boarding with experts